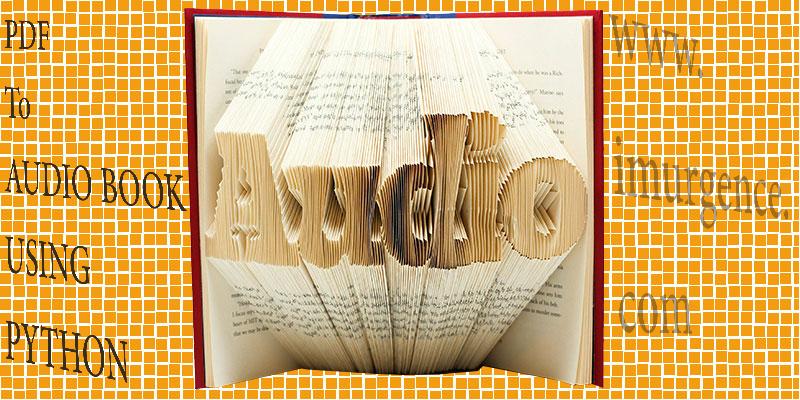
How to create a audio book using Python ?
To create an audio book we need to first install some packages and also the file must be in PDF format and placed in that folder where your python code is present for easy access to the file. You may face a bit of challenge if you are using a PDF file created from application like WPS. If the file content is not getting converted into audio, use a open source PDF convertor and test the code again. For ease of testing the code we are keeping a copy of the PDF file which we have used. Click on the link Creating your first machine learning project.
Once downloaded, place this in your working directory.
we use two libraries in our code.
pyttsx3: Used for converting our text to speech. It is a text-to-speech conversion library in Python. Unlike alternative libraries, it works offline and is compatible with both Python 2 and 3. 2.
PyPDF2: Use for retrieving the data from the PDF file and many more. A Pure-Python library built as a PDF toolkit. Functionalities include :
extract pdf doc information (title, author, …)
split pdf page by page
merge pdf page by page
crop pdf pages
merge multiple pdf documents into a single document
encrypt and decrypt PDF files
By being Pure-Python, it should run on any Python platform without any dependencies on external libraries. It can also work entirely on StringIO objects rather than file streams, allowing for PDF manipulation in memory. It is therefore a useful tool for websites that manage or manipulate PDF's.
Import the Packages
import pyttsx3
import PyPDF2
book = open('Creating your first machine learning project.pdf','rb')
# replace the file name with your pdf file name
pdfReader = PyPDF2.PdfFileReader(book)
# Here, we create an object of PdfFileReader class of the PyPDF2 module and pass the pdf file object & get a pdf reader object.
pages = pdfReader.numPages
# numPages property gives the number of pages in the pdf file
speaker = pyttsx3.init()
# pyttsx3. init() factory function is used to get a reference to a pyttsx3.
for i in range(pages):
page = pdfReader.getPage(i)
# Retrieves a page by the number from this PDF file
text = page.extractText()
# extractText() is used to extract text from the pdf page.
page_no = i + 1
tell = ('Page number is',page_no)
speaker.say(tell)
speaker.say(text)
speaker.runAndWait()
# This function will make the speech audible in the system, if you don't write this command then the speech will not be audible to you.
Next Step
This implementation doesn't allow you to stop the audio book using any user action like a keyboard press or so on. As a next improvement you can look at loop interruption for stopping the code execution. Also here we have considered only a PDF document, you can look at other file extensions for audio conversion.
To create an audio book we need to first install some packages and also the file must be in PDF format and placed in that folder where your python code is present for easy access to the file. You may face a bit of challenge if you are using a PDF file created from application like WPS. If the file content is not getting converted into audio, use a open source PDF convertor and test the code again. For ease of testing the code we are keeping a copy of the PDF file which we have used. Click on the link Creating your first machine learning project.
Once downloaded, place this in your working directory.
we use two libraries in our code.
- pyttsx3: Used for converting our text to speech. It is a text-to-speech conversion library in Python. Unlike alternative libraries, it works offline and is compatible with both Python 2 and 3. 2.
- PyPDF2: Use for retrieving the data from the PDF file and many more. A Pure-Python library built as a PDF toolkit. Functionalities include :
- extract pdf doc information (title, author, …)
- split pdf page by page
- merge pdf page by page
- crop pdf pages
- merge multiple pdf documents into a single document
- encrypt and decrypt PDF files
By being Pure-Python, it should run on any Python platform without any dependencies on external libraries. It can also work entirely on StringIO objects rather than file streams, allowing for PDF manipulation in memory. It is therefore a useful tool for websites that manage or manipulate PDF's.
Import the Packages
import pyttsx3
import PyPDF2
book = open('Creating your first machine learning project.pdf','rb')
# replace the file name with your pdf file name
pdfReader = PyPDF2.PdfFileReader(book)
# Here, we create an object of PdfFileReader class of the PyPDF2 module and pass the pdf file object & get a pdf reader object.
pages = pdfReader.numPages
# numPages property gives the number of pages in the pdf file
speaker = pyttsx3.init()
# pyttsx3. init() factory function is used to get a reference to a pyttsx3.
for i in range(pages):
page = pdfReader.getPage(i)
# Retrieves a page by the number from this PDF file
text = page.extractText()
# extractText() is used to extract text from the pdf page.
page_no = i + 1
tell = ('Page number is',page_no)
speaker.say(tell)
speaker.say(text)
speaker.runAndWait()
# This function will make the speech audible in the system, if you don't write this command then the speech will not be audible to you.
Next Step
This implementation doesn't allow you to stop the audio book using any user action like a keyboard press or so on. As a next improvement you can look at loop interruption for stopping the code execution. Also here we have considered only a PDF document, you can look at other file extensions for audio conversion.
Write A Public Review